服务注册中心Consul
什么是Consul?
Consul是HashiCorp公司推出的开源工具,用于实现分布式系统的服务发现与配置。 Consul是分布式的、高可用的、可横向扩展的。它具备以下特性 : service discovery:consul通过DNS或者HTTP接口使服务注册和服务发现变的很容易,一些外部服务,例如saas提供的也可以一样注册。 health checking:健康检测使consul可以快速的告警在集群中的操作。和服务发现的集成,可以防止服务转发到故障的服务上面。 key/value storage:一个用来存储动态配置的系统。提供简单的HTTP接口,可以在任何地方操作。 multi-datacenter:无需复杂的配置,即可支持任意数量的区域。
Consul安装
下载Consul
访问官网:https://www.consul.io/downloads ;根据你需要部署的环境选择版本;
解压
解压后得到Consul可执行文件,可以通过环境变量或者拷贝到/usr/local/bin 来在任意位置执行该程序。
检查是否安装
huzd@huzd-MacBook-Pro Downloads % ./consul --help
Usage: consul [--version] [--help] <command> [<args>]
Available commands are:
acl Interact with Consul's ACLs
agent Runs a Consul agent
catalog Interact with the catalog
config Interact with Consul's Centralized Configurations
connect Interact with Consul Connect
debug Records a debugging archive for operators
event Fire a new event
exec Executes a command on Consul nodes
force-leave Forces a member of the cluster to enter the "left" state
info Provides debugging information for operators.
intention Interact with Connect service intentions
join Tell Consul agent to join cluster
keygen Generates a new encryption key
keyring Manages gossip layer encryption keys
kv Interact with the key-value store
leave Gracefully leaves the Consul cluster and shuts down
lock Execute a command holding a lock
login Login to Consul using an auth method
logout Destroy a Consul token created with login
maint Controls node or service maintenance mode
members Lists the members of a Consul cluster
monitor Stream logs from a Consul agent
operator Provides cluster-level tools for Consul operators
reload Triggers the agent to reload configuration files
rtt Estimates network round trip time between nodes
services Interact with services
snapshot Saves, restores and inspects snapshots of Consul server state
tls Builtin helpers for creating CAs and certificates
validate Validate config files/directories
version Prints the Consul version
watch Watch for changes in Consul
启动
执行命令:consul agent -dev
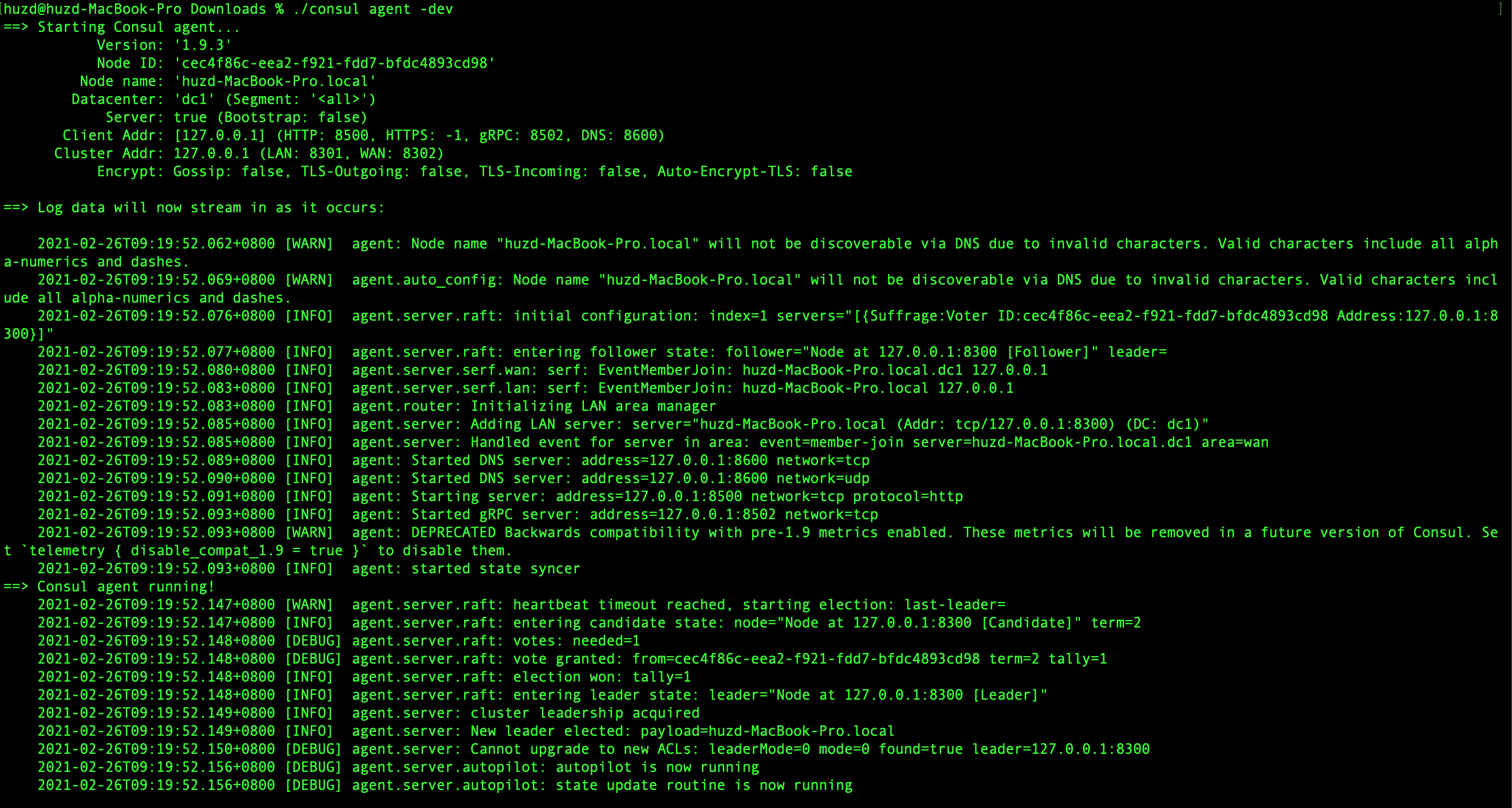
说明:
- -dev(该节点的启动不能用于生产环境,因为该模式下不会持久化任何状态),该启动模式仅仅是为了快速便捷的启动单节点consul
- 该节点处于server模式
- 该节点是leader
- 该节点是一个健康节点
查看Consul节点信息
命令行形式
huzd@huzd-MacBook-Pro Downloads % ./consul members
Node Address Status Type Build Protocol DC Segment
huzd-MacBook-Pro.local 127.0.0.1:8301 alive server 1.9.3 2 dc1 <all>
说明:
- Address:节点地址
- Status:alive表示节点健康
- Type:server运行状态是server状态
- DC:dc1表示该节点属于DataCenter1
注意:
- members命令的输出是基于gossip协议的,并且是最终一致的(也就是说,某一个时刻你去运用该命令查到的consul节点的状态信息可能是有误的)
后台管理界面
访问http://localhost:8500

SpringCloud 整合 Consul
创建基于Consul的服务提供者
创建maven项目:
创建 cloud-provider-payment-consul-8006
修改pom.xml:
<!-- 添加consul服务发现jar -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-consul-discovery</artifactId>
</dependency>
修改application.yml:
添加Consul相关配置
spring:
cloud:
consul:
host: localhost
port: 8500
discovery:
service-name: ${spring.application.name}
application:
name: cloud-provider-payment
添加业务逻辑:
添加一个测试方法:url:/payment/consul
@Controller
@RequestMapping("/payment")
@Slf4j
public class PaymentController {
@Resource
PaymentService paymentService;
@Value("${server.port}")
Integer port;
@GetMapping("/consul")
@ResponseBody
public Object zk() {
return "This is from Payment Service of Register :"+port + ",Random String:"+ UUID.randomUUID();
}
}
启动服务:
启动类:
@SpringBootApplication
@EnableDiscoveryClient
public class CloudProviderPayment8006Application {
public static void main(String[] args) {
SpringApplication.run(CloudProviderPayment8006Application.class,args);
}
}
启动关键日志:
2021-02-26 09:50:04.014 INFO 1715 --- [ restartedMain] o.s.c.c.s.ConsulServiceRegistry : Registering service with consul: NewService{id='cloud-provider-payment-8006', name='cloud-provider-payment', tags=[secure=false], address='130.30.3.224', meta={}, port=8006, enableTagOverride=null, check=Check{script='null', dockerContainerID='null', shell='null', interval='10s', ttl='null', http='http://130.30.3.224:8006/actuator/health', method='null', header={}, tcp='null', timeout='null', deregisterCriticalServiceAfter='null', tlsSkipVerify=null, status='null', grpc='null', grpcUseTLS=null}, checks=null}
查看后台管理:
刷新consul后台管理界面:
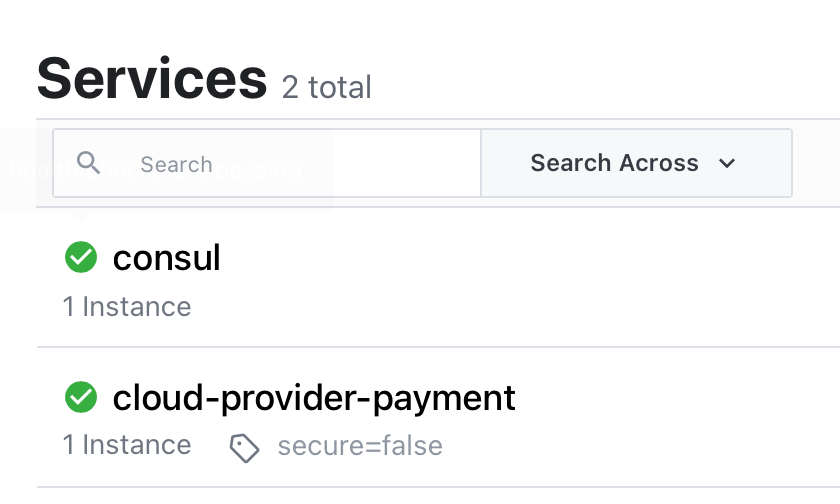
详情页:
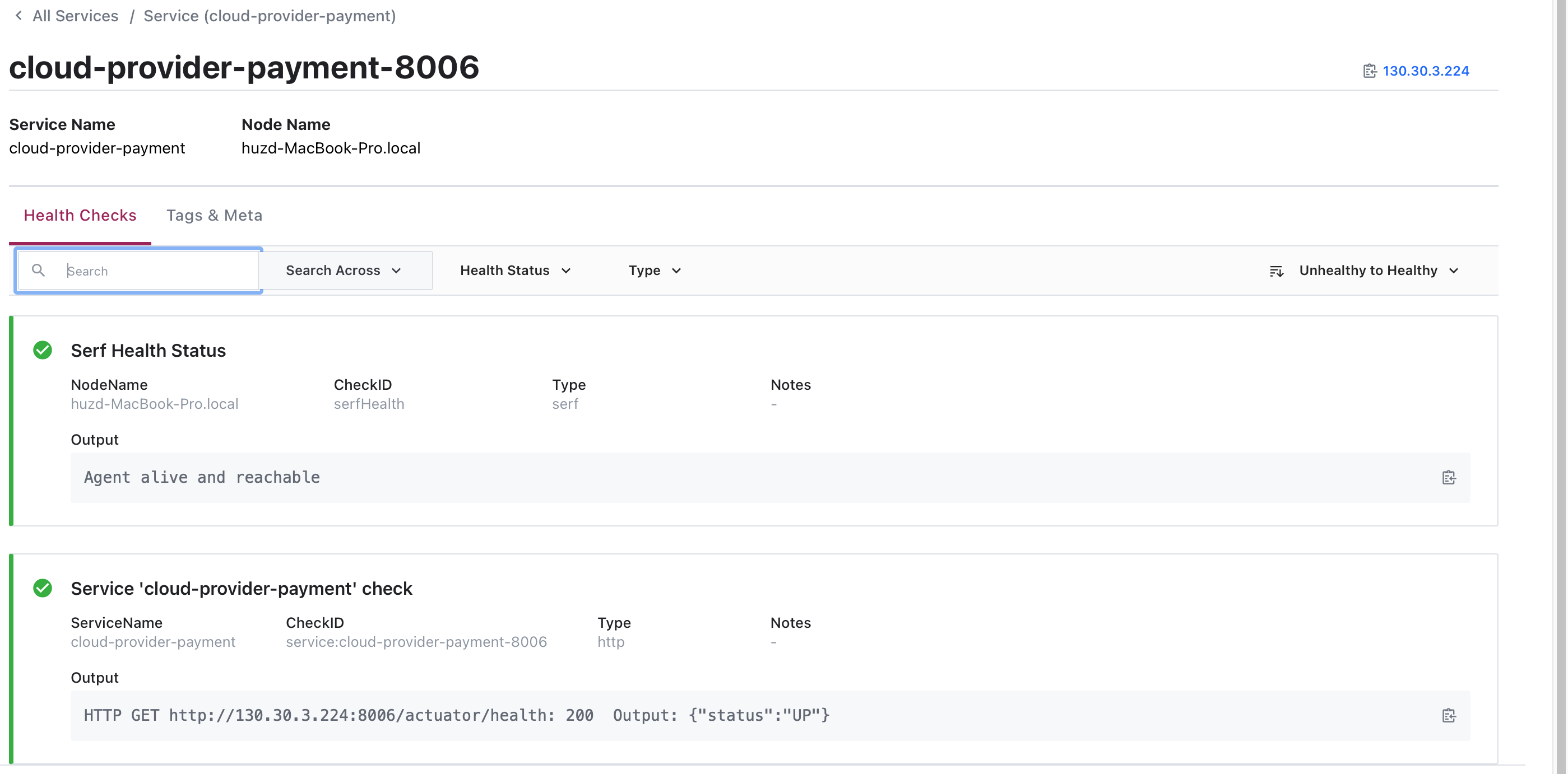
访问服务:
创建基于Consul的服务消费者
创建maven项目:
创建 cloud-consumer-order-consul-80
修改pom.xml:
<!-- 添加consul服务发现jar;其他省略 -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-consul-discovery</artifactId>
</dependency>
修改application.yml:
添加Consul相关配置
server:
port: 80
spring:
application:
name: cloud-consumer-order-consul-80
cloud:
consul:
host: 127.0.0.1
port: 8500
discovery:
service-name: ${spring.application.name}
添加业务逻辑:
添加一个测试方法:url:/consumer/payment/consul
@Controller
@RequestMapping("/consumer")
@Slf4j
public class OrderController {
public static final String PAYMENT_URL = "http://cloud-provider-payment/";
@Resource
RestTemplate restTemplate;
@GetMapping("/payment/consul")
@ResponseBody
public String consul(){
System.out.println("请求地址:"+PAYMENT_URL+"payment/consul");
return restTemplate.getForObject(PAYMENT_URL+"/payment/consul",String.class);
}
}
启动服务:
启动类:
@SpringBootApplication
@EnableDiscoveryClient
public class CloudConsumerOrderConsulApplication {
public static void main(String[] args) {
SpringApplication.run(CloudConsumerOrderConsulApplication.class,args);
}
}
启动关键日志:
2021-02-26 10:05:27.772 INFO 1923 --- [ restartedMain] o.s.c.c.s.ConsulServiceRegistry : Registering service with consul: NewService{id='cloud-consumer-order-consul-80-80', name='cloud-consumer-order-consul-80', tags=[secure=false], address='130.30.3.224', meta={}, port=80, enableTagOverride=null, check=Check{script='null', dockerContainerID='null', shell='null', interval='10s', ttl='null', http='http://130.30.3.224:80/actuator/health', method='null', header={}, tcp='null', timeout='null', deregisterCriticalServiceAfter='null', tlsSkipVerify=null, status='null', grpc='null', grpcUseTLS=null}, checks=null}
查看后台管理:
刷新consul后台管理界面:
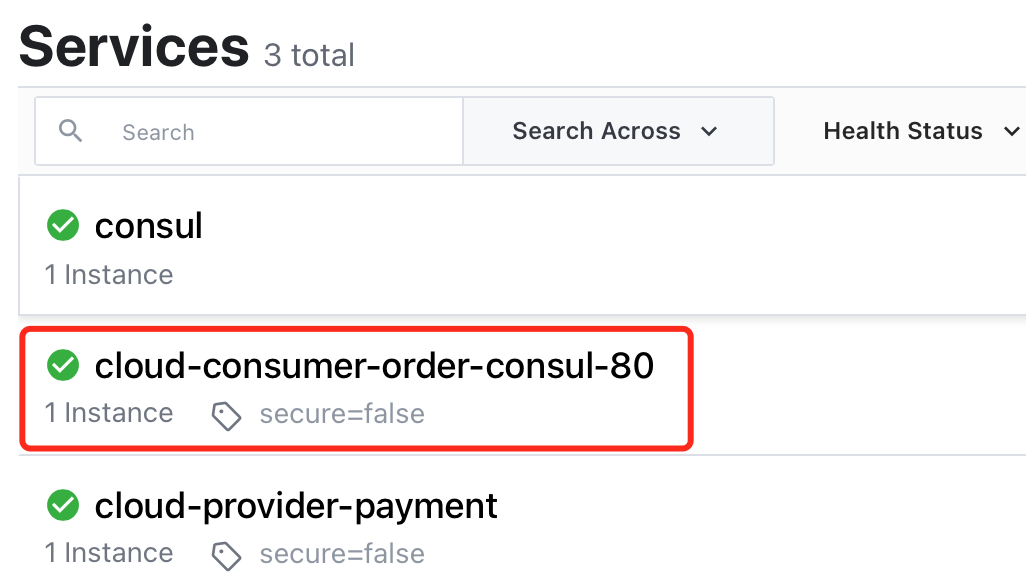
详情页:
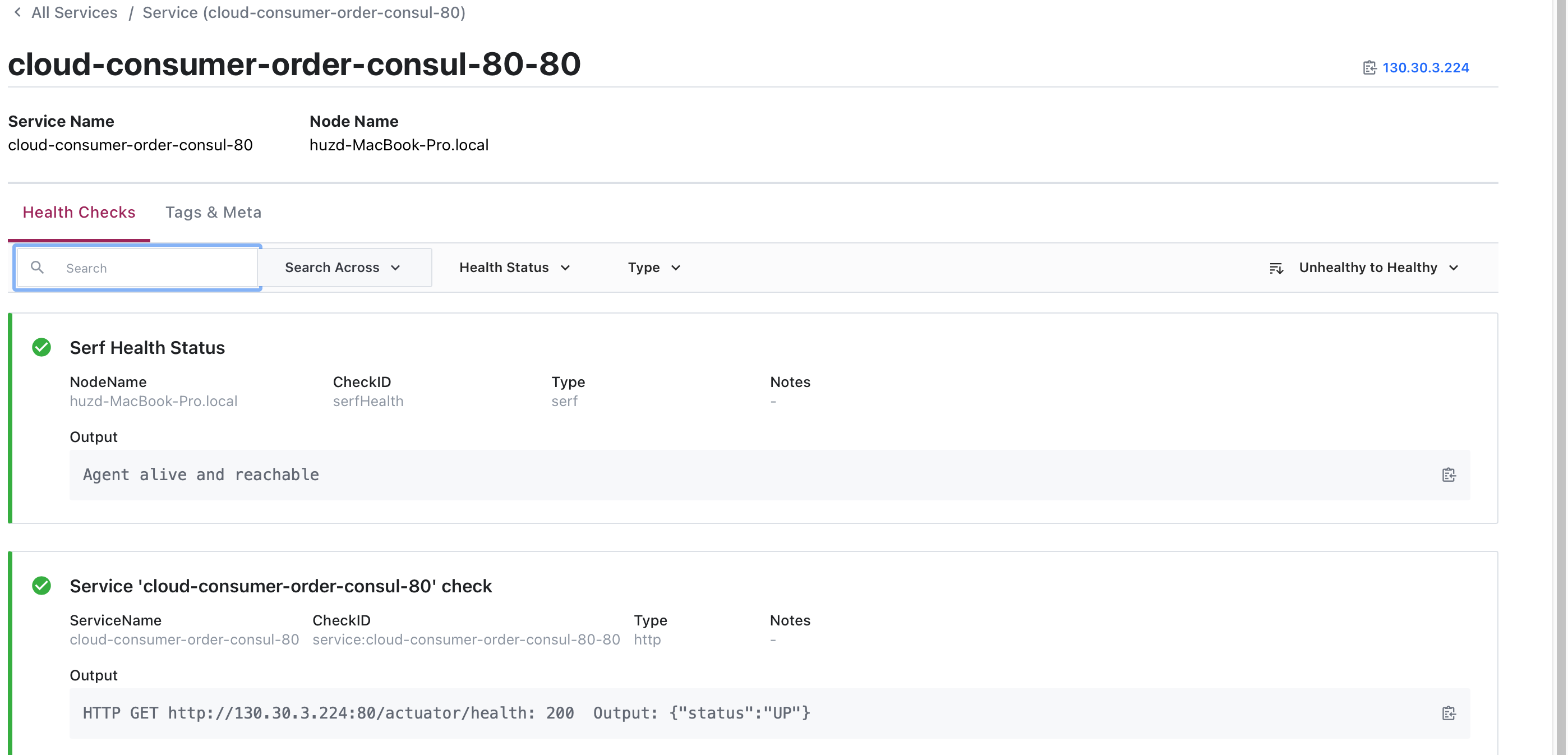
访问服务:

本文由 huzd 创作,采用 知识共享署名4.0 国际许可协议进行许可本站文章除注明转载/出处外,均为本站原创或翻译,转载前请务必署名最后编辑时间
为:
2021/02/26 10:42