SpringCloud Config
Config简介
Config项目是一个解决分布式系统的配置管理方案。它包含了Client和Server两个部分,server提供配置文件的存储、以接口的形式将配置文件的内容提供出去,client通过接口获取数据、并依据此数据初始化自己的应用。
Config为各个微服务应用提供了一个中心化的外部配置。
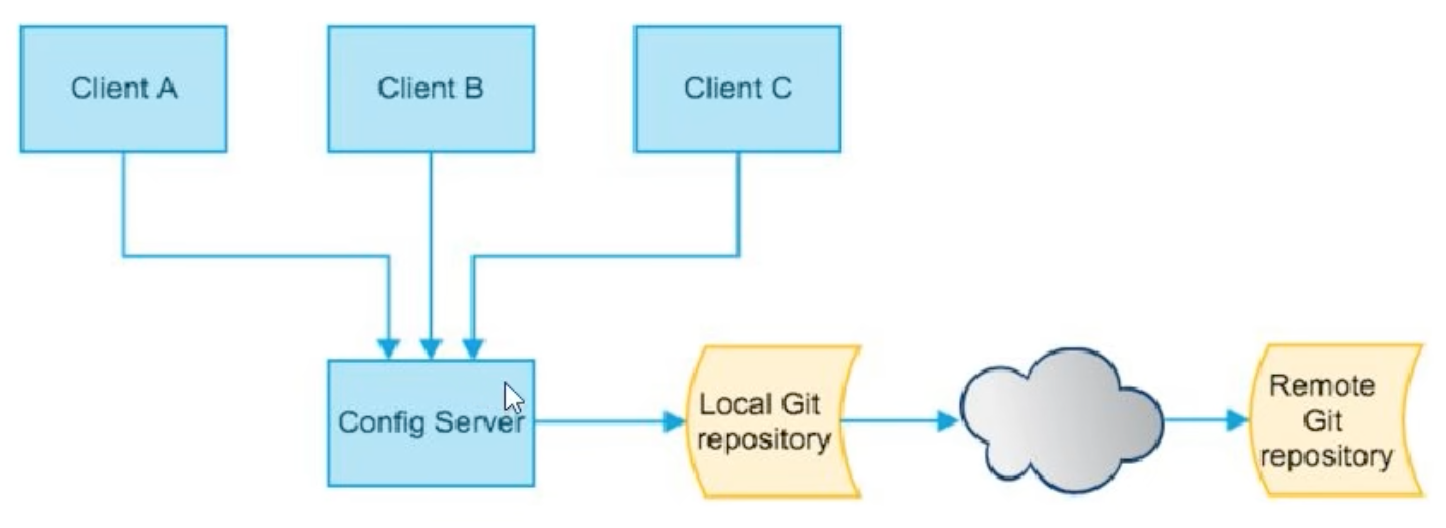
- Server:称之为分布式配置中心,它是一个独立的微服务应用。用来连接配置服务器并为客户端提供获取配置信息、加密\解密信息等的接口。
- client:客户端是通过指定的配置中心来管理应用资源以及与业务相关的配置内容,并在启动的时候从配置中心获取和加载配置信息配置服务器,默认采用Git来存储信息,这样有助于对环境配置信息进行版本管理,
Config功能
- 集中管理配置文件;
- 不同环境不同配置,动态化的配置更新,分环境部署比如dev/test/prod/beta/release
- 运行期间动态调整配置,无需在每个服务部署机器上编写配置文件,服务会向配置中心统一拉取自己的配置信息。
- 当配置发生变动时,服务不需要重启即可感知到配置的变化并应用型的配置。
- 将配置信息以REST借口的形式暴露
Config 服务端实操案例
新建仓库
在Github或者Gitlab上创建一个新的仓库。
http://git.si-tech.com.cn:9002/huzd/springcloud-config.git
因为在公司内网环境Github被墙了;无法直接访问;这里我自己使用公司的gitlab,两者原理一致;注意替换地址就好。
Git配置文件准备
- 创建项目
在idea中创建一个新的项目,File -> New -> Project from Version Control...
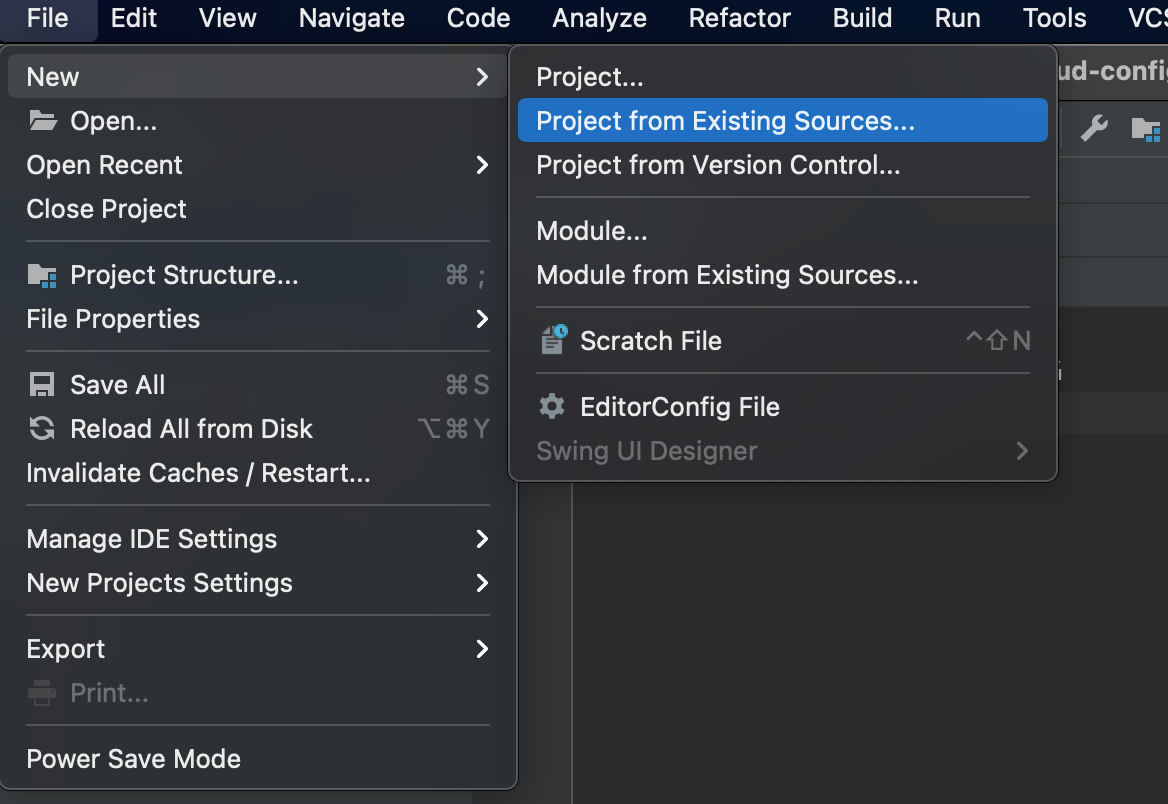
- 填写配置
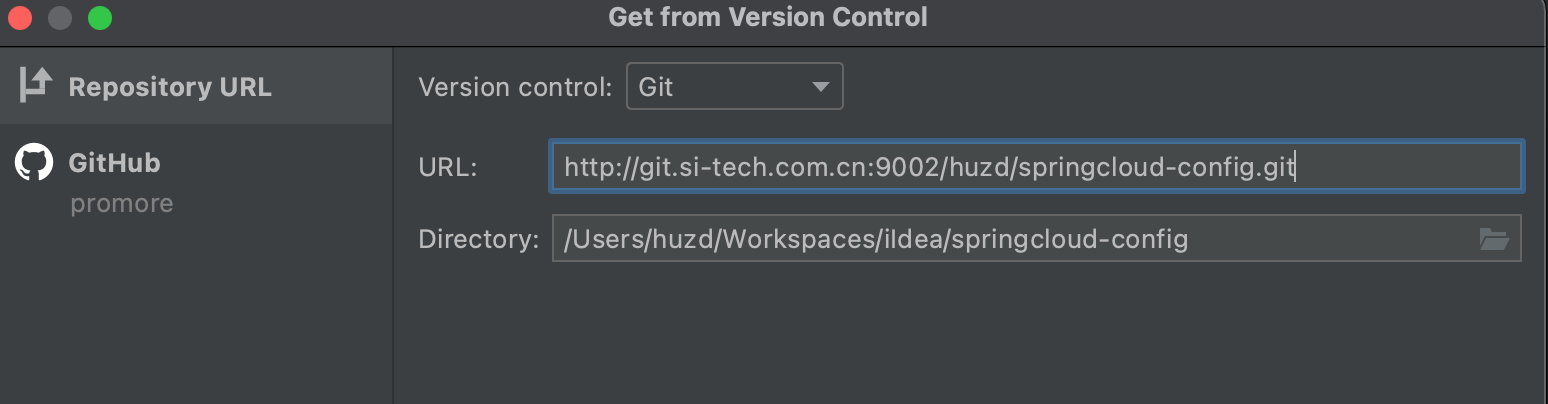
-
创建三个配置文件
- config-dev.yml
server: port: 3355
- config-prod.yml
server: port: 3366
- config-test.yml
server: port: 3377
-
提交到gitlab(或者Github)远程库
先commit,然后push到远程仓库
创建Maven项目
创建命为:cloud-config-center-3344 的项目
修改pom.xml文件
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-server</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
创建application.yml
server:
port: 3344
spring:
application:
name: cloud-config-center
cloud:
config:
server:
git:
uri: http://git.si-tech.com.cn:9002/huzd/springcloud-config.git #填写配置文件所在的git仓库地址
search-paths:
- springcloud-config #填写git项目名称
username: huzd@si-tech.com.cn #git 或者 gitlab 的用户名
password: ******123 #密码
skip-ssl-validation: true
label: master #分支
eureka:
client:
service-url:
defaultZone: http://eureka7001.com:7001/eureka,http://eureka7002.com:7002/eureka
创建启动类
@SpringBootApplication
@EnableConfigServer
@EnableEurekaClient
public class CloudConfigCenter3344Application {
public static void main(String[] args) {
SpringApplication.run(CloudConfigCenter3344Application.class,args);
}
}
启动测试
访问地址:http://config-3344.com:3344/master/config-prod.yml
结果如下:
server:
port: 3366
配置读取规则
SpringCloud Config 提供了五种路径的规则。
/{application}/{profile}[/{label}]
#这种方式返回的结果如下:
#{"name":"config","profiles":["prod"],"label":"master","version":"e341b29b3de99a16303b11ae15a919accb0c2b49","state":null,"propertySources":[{"name":"http://git.si-tech.com.cn:9002/huzd/springcloud-config.git/config-prod.yml","source":{"server.port":3366}}]}
# 需要自己再去解析。
/{application}-{profile}.yml
#这时候默认使用application.yml里面的label - master
/{label}/{application}-{profile}.yml
#label 为git版本的分支,在联系的时候可以创建一个dev分支;并且添加属性name:dev 切换访问路径检查效果。
/{application}-{profile}.properties
#同上类比
/{label}/{application}-{profile}.properties
#同上类比
注意事项:
访问不存在的配置路径时例如:http://config-3344.com:3344/config-test2.yml,返回{}。
Config客户端实操案例
创建maven项目
创建名为:cloud-config-client-3355
修改pom.xml
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
创建bootstrap.yml
server:
port: 3355
spring:
application:
name: config-client
cloud:
config:
label: master
name: config
profile: dev
uri: http://localhost:3344
eureka:
client:
service-url:
defaultZone: http://eureka7001.com:7001/eureka,http://eureka7002.com:7002/eureka
创建启动类
@SpringBootApplication
@EnableEurekaClient
public class CloudConfigClient3355Application {
public static void main(String[] args) {
SpringApplication.run(CloudConfigClient3355Application.class,args);
}
}
测试
访问:http://localhost:3355/configInfo

gitlab配置文件为:
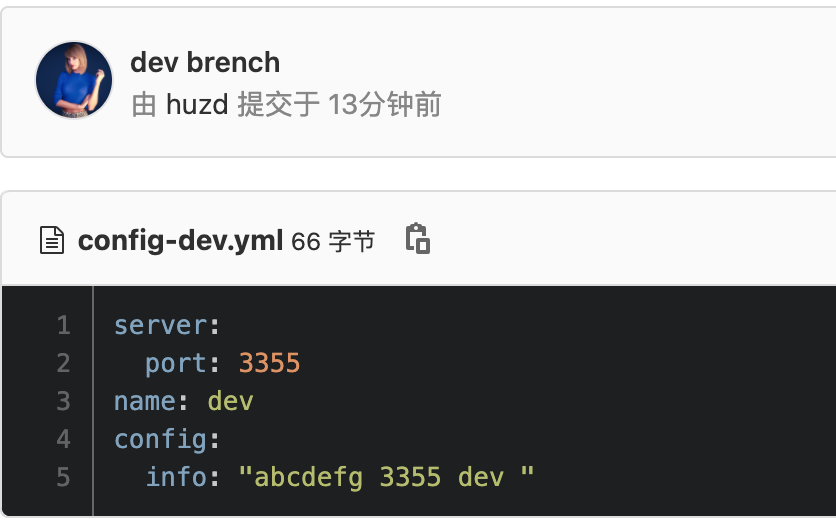
总结
配置文件
- application.yml 是应用级配置文件
- bootstrap.yml 是系统级配置文件,优先级更高
SpringCloud 会创建一个 Bootstrap Context,作为Spring应用的 Application Context的父上下文。初始化时Bootstrap Context 负责从外部源加载配置属性并解析配置。这两个上下文共享一个从外部获取的Environment。
Bootstrap 属性有高优先级,默认情况下,它们不会被本地配置覆盖。Bootstrap Context和Applicaton Context 有着不同的约定,所以新增一个bootstrap.yml文件,保障 Bootstrap Context 和 Application Context 配置的分离。
所以:==在创建config客户端时,需要将application.yml 改成 bootstrap.yml。==
手动刷新同步配置
上面的实例中当Github的版本更新后;SpringCloud Config可以获取最新的更新配置;但是客户端3355依然是老的配置;我们需要对3355客户端做如下更改来是想手动刷新3355的配置。
客户端添加Actuator
在3355的pom.xml中添加如下内容:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
修改YML
在3355的yaml配置文件中添加如下内容:
management:
endpoints:
web:
exposure:
include: "*"
修改Controller
@RestController
@RefreshScope //添加注解
public class ConfigController {
@Value("${config.info}")
private String configInfo;
@GetMapping("/configInfo")
public String getConfigInfo(){
return configInfo;
}
}
启动测试
测试步骤:
- 在Gitlab修改config-dev.yml文件;
- 访问http://localhost:3344/master/config-dev.yml 可以立刻看到gitlab更新的内容;
- 访问http://localhost:3355/configInfo 发现内容还是未更新;
- 在命令行执行:curl -X POST "http://localhost:3355/actuator/refresh”
- 再次访问访问http://localhost:3355/configInfo 发现内容已经更新。
总结:
目前虽然实现了配置文件的GIT拉取;但还无法做到自动更新。后面的章节将结合服务总线BUS完成自动更新。
本文由 huzd 创作,采用 知识共享署名4.0 国际许可协议进行许可本站文章除注明转载/出处外,均为本站原创或翻译,转载前请务必署名最后编辑时间
为:
2021/03/16 10:46